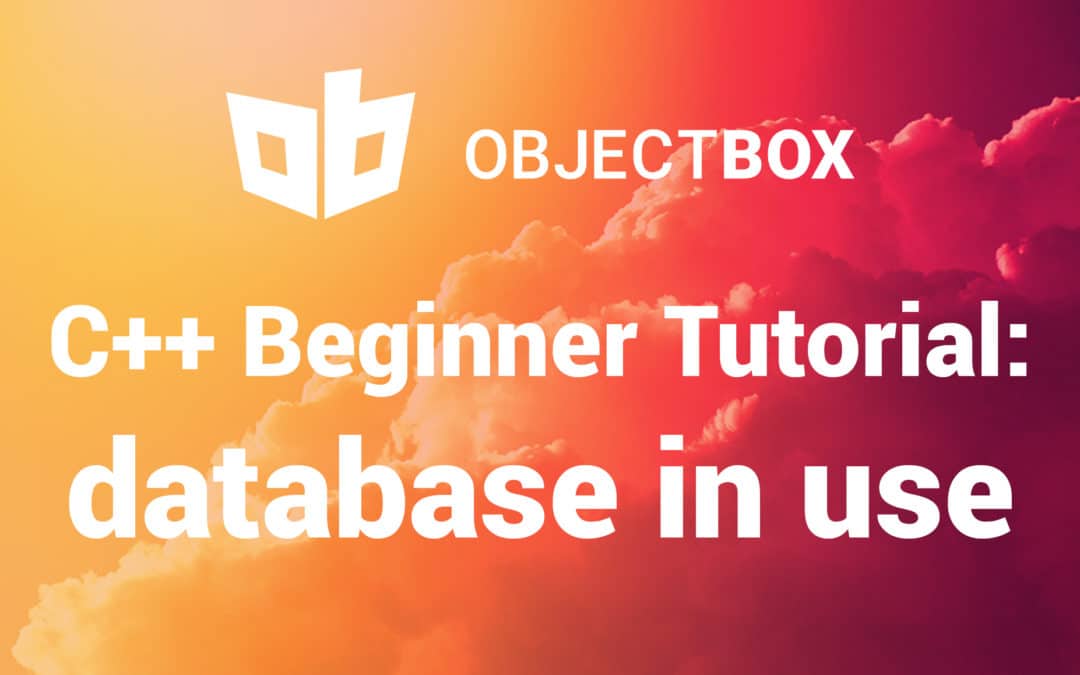
Beginner C++ Database Tutorial: How to use ObjectBox
Introduction
As a direct follow up from the ObjectBox database installation tutorial, today we’ll code a simple C++ example app to show how the database can be used. Before starting to program, let’s briefly overview what we want to achieve with this tutorial and what is the best way to work through it.
Overview of the app we want to build
In short, we will make a console calculator app with an option to save results into memory. These will be stored as objects of the Number class. Every Number will also have an ID for easy reference in future calculations. Apart from the function to make calculations, we will create a function to enter memory. It will list all the database entries and have an option to clear memory. By coding all of this, we will make use of such standard ObjectBox operations as put, get, getAll and removeAll.
Our program will consist of seven files:
- the FlatBuffers schema file, that defines the model of a class we want to store in the database
- the header file, for class function definitions
- the source file, for function implementation
- the four files with objectbox binding code that will be created by objectbox-generator
How to use this tutorial
While looking at coding examples is useful in many cases, the best way to learn such a practical skill like programming is to solve problems independently. This is why we included an exercise for each step. You are encouraged to make the effort and do each of them, even if you don’t know the answer straight away. Only move to the next step after you test each part of your program and make sure that everything works as intended. Ideally, you should only use the code snippets presented here to check yourself or look for hints when you feel stuck. Bear in mind that sometimes there might be several different ways to achieve the same results. So if something that we ask you to do in this tutorial doesn’t work for you, try to come up with your own solution.
How to create the FlatBuffers file?
First, we’ll create the FlatBuffers schema (.fbs) for our app. This is required for the objectbox-generator to generate binding code that will allow us to use the ObjectBox library in our project.
The FlatBuffers schema consists of a table, which defines the object we want to store in the database, and the properties of this object. Each property consists of a name and a type. We want to keep our example very simple, so just two properties is enough.
- To replicate a calculator’s memory, we want ObjectBox to store some numbers. We can define the Number object by giving the table a corresponding name.
- Inside the table, we want to have two properties: id and contents. The contents of each Number object is the number itself (double), while id is an ulong that our program will assign to each of them for easy identification.
Exercise: create a file called numbers.fbs and define the table in the format
1 2 3 |
table Name { property_name: type; } |
Reveal code
1 2 3 4 |
table Number { id: ulong; contents: double; } |
Generating binding code
Now that the FlatBuffers file is ready, we can generate the binding code. To do this, run the objectbox-generator for our FlatBuffers file:
1 |
objectbox-generator -cpp numbers.fbs |
The following files will be generated:
- objectbox-model.h
- objectbox-model.json
- numbers.obx.hpp
- numbers.obx.cpp
The header file
This is where the main chunk of our code will be. It will contain the Calculator class and all the function definitions.
- Start by including the three ObjectBox header files: objectbox.hpp, objectbox-model.h and numbers.obx.hpp. Our whole program will be based on one class, called Calculator. It should only have two private members: Store and Box. Store is a reference to the database and will manage Boxes. Each Box stores objects of a particular class. In this example, we only need one Box. Let’s call it numberBox, as it will store Numbers that we want to save in the memory of our calculator.
Exercise: create a file called calculator.hpp and define the Calculator class with two private members: reference to the obx library member Store and a Box of Numbers.
Reveal code
1 2 3 4 |
class Calculator { obx::Store& store; obx::Box<Number> numberBox; } |
2. After the constructor, we define the run function. It will be responsible for the menu of our program. There should be two main options: to perform calculations and enter memory. As discussed above, we want this app to do two things: perform calculations and show memory. We’ll define these as separate functions, called Calculate and Memory. The first one is quite standard, so we won’t go into a detailed explanation here. The only thing you should keep in mind is that we need to account for the case when the user wants to operate on a memory item. To deal with this, we’ll process input in a function called processInput.
Exercise: define the parametrised constructor which takes a reference to Store as a parameter. Then define the run and Calculate functions.
Reveal code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
public: Calculator(obx::Store& obxStore) : store(obxStore), numberBox(obxStore) {} int run(){ std::string input; std::cout << "Welcome to the example calculator app." << std::endl; while(true) { std::cout << std::endl << "Available commands:" << std::endl << " calc - make a calculation;" << std::endl << " memory - see stored numbers;" << std::endl << " exit." << std::endl; std::getline(std::cin, input); if(input == "calc") { Calculate(); continue; } else if(input == "memory") { Memory(); continue; } else if(input == "exit") { std::exit(0); } else { std::cerr << "Unknown command." << std::endl; fflush(stderr); continue; } } } void Calculate() { std::string input; double x; double y; char op; double result; std::cout << "Enter an operation in the format x [+,-,*,/] y: "; while (std::getline(std::cin, input)) { if(input == "back"){ break;} bool valid = processInput(input, x, op, y); if(valid == false) { continue;} switch(op) { case '+': result = x + y; break; case '-': result = x - y; break; case '*': result = x * y; break; case '/': result = x / y; break; } } } std::cout << "Result: " << x << " " << op << " " << y << " = " << result << std::endl; |
3. The final part of this function is for saving results into memory. We start by asking the user if they want to do that. If the answer is positive, we create a new instance of Number and set the most recent result as a value of its contents. To save our object in the database, we can operate with put(object) on our Box. put is one of the standard ObjectBox operations, which is used for creating new objects and overwriting existing ones.
Exercise: create an option to store the result in memory, making use of the ObjectBox put operation.
Reveal code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
std::cout << "Save this number [y/n]? "; while(true){ std::getline(std::cin, input); if(input == "y"){ Number object{}; object.contents = result; numberBox.put(object); std::cout << "New number in memory: " << object.contents << ", ID: " << object.id << std::endl; break; } else if (input == "n") { break; } else { std::cerr << "Unknown command. Try again: "; fflush(stderr); continue; } } |
4. Next, we should define processInput, which will read input as a string and check whether it has the right format. Now, to make it recognise the memory items, we have to come up with a standard format for these. Remember, we defined an ID property for our Numbers. Every number in our database has an ID, so we can refer to them as, e.g. m1, m2, m3 etc. To read the numbers from memory, we can make use of the get(obx_id) operation. It returns a unique pointer to the corresponding Number, whose contents we need to access and use as our operand.
Exercise: define the processInput function, which detects when something like m1 was used as an operand and updates x, y, and op according to the input.
Reveal code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
bool processInput(const std::string& input, double& x, char& op, double& y) { std::vector<std::reference_wrapper<double>> outNumbers; outNumbers.push_back(x); outNumbers.push_back(y); std::vector<std::string> inputStrings; std::string strX; std::string strY; std::istringstream inputStream(input); inputStream.str(input); inputStream >> strX >> op >> strY; inputStrings.push_back(strX); inputStrings.push_back(strY); //Iterate over the two numbers from input, retrieving them from memory if needed for(int i = 0; i < 2; i++) { try { if(inputStrings[i][0] == 'm') { inputStrings[i] = inputStrings[i].substr(1); obx_id id = std::stoull(inputStrings[i]); std::unique_ptr<Number> num = numberBox.get(id); outNumbers[i].get() = num->contents; } else { outNumbers[i].get() = std::stod(inputStrings[i]); } } catch(std::exception& e) { std::cout << "Invalid input. Try again: "; return false; } } return true; } |
5. The last function in our header file will be Memory. It should list all the numbers contained in the database and have an option to clear data. We can read all the database entries by calling the getAll ObjectBox operator. It returns a vector of unique pointers. To clear memory, you can simply operate with removeAll on our Box.
Exercise: define the Memory function, which lists all the memory items, and can delete all of them by request.
Reveal code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
void Memory() { std::string input; std::vector<std::unique_ptr<Number>> list; list = numberBox.getAll(); for(const auto& number : list) { std::cout << number->id << " " << number->contents << std::endl; } std::cout << "Enter clear to delete all or back to return to menu. " << std::endl; while(std::getline(std::cin, input)) { if(input == "clear") { numberBox.removeAll(); std::cout << "Data deleted." << std::endl; break; } else if (input == "back") { break; } else { std::cerr << "Unknown command." << input << std::endl; fflush(stderr); continue; } } } |
The source file
To tie everything together, we create a source (.cpp) file. It should contain only the main function that initialises the objectbox model, creates an instance of the Calculator app, and runs it. To create the ObjectBox model, use
1 |
obx::Store::Options options(create_obx_model()) |
then passing options as a parameter when you initialise the Store.
Exercise: create the source file
Reveal code
1 2 3 4 5 6 7 8 9 |
#include "example.hpp" int main() { obx::Store::Options options(create_obx_model()); obx::Store store(options); Calculator app(store); return app.run(); } |
Final notes
Now you can finally compile and run your application. At this point, a good exercise would be to try and add some more functionality to this project. Check out the ObjectBox C++ documentation to learn more about the available operations.
After you’ve mastered ObjectBox DB, why not try ObjectBox Sync? Here is another tutorial from us, showing how easily you can sync between different instances of your cross platform app.
Other than that, if you spot any errors in this tutorial or if anything is unclear, please come back to us. We are happy to hear your thoughts.